I’m building a Figma plugin to create and manage variables and style easily. At first, everything was smooth— I had a simple structure, and adding features felt effortless. But as the project grew, things started getting messy. Managing state became a nightmare.
I was using Context API with reducers, thinking it was the best approach. But soon, my code was drowning in providers, actions, and reducers spread across multiple files. Every small change felt like a battle. Debugging was a headache. I knew there had to be a better way.
That’s when I found Zustand. I decided to give it a shot, and within hours, I was hooked. What used to take dozens of files now needed just a few simple stores. My code was cleaner, easier to manage, and my development speed shot up.
Let me show you why Zustand completely changed how I handle state in React!
A Simple Counter Example
Before Zustand (Using Context API)
// CounterContext.jsx
import React, { createContext, useContext, useReducer } from 'react'
// 1. Create context
const CounterContext = createContext()
// 2. Define reducer
function counterReducer(state, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 }
case 'DECREMENT':
return { count: state.count - 1 }
case 'RESET':
return { count: 0 }
default:
return state
}
}
// 3. Create provider
export function CounterProvider({ children }) {
const [state, dispatch] = useReducer(counterReducer, { count: 0 })
return (
<CounterContext.Provider value={{ state, dispatch }}>
{children}
</CounterContext.Provider>
)
}
// 4. Create custom hook
export function useCounter() {
const context = useContext(CounterContext)
if (!context) {
throw new Error('useCounter must be used within CounterProvider')
}
return context
}
Then in your component:
// Counter.jsx with Context
import { useCounter } from './CounterContext'
function Counter() {
const { state, dispatch } = useCounter()
return (
<div>
<h1>Count: {state.count}</h1>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>+</button>
<button onClick={() => dispatch({ type: 'DECREMENT' })}>-</button>
<button onClick={() => dispatch({ type: 'RESET' })}>Reset</button>
</div>
)
}
And you'd still need to wrap your app with:
<CounterProvider>
<App />
</CounterProvider>
### Using Zustand
``` typescript
// store.js
import { create } from 'zustand'
// Create a store
const useCounterStore = create((set) => ({
// State
count: 0,
// Actions
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
reset: () => set({ count: 0 }),
}))
export default useCounterStore
That's it! Now you can use this store in any component:
// Counter.jsx
import useCounterStore from './store'
function Counter() {
// Extract just what you need from the store
const { count, increment, decrement, reset } = useCounterStore()
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
<button onClick={reset}>Reset</button>
</div>
)
}
- No Providers - Just create your store and use it anywhere
- No Boilerplate - No need for action types, reducers, or dispatch
- Simple Syntax - State and actions in one place
- Selective Updates - Components only re-render when their specific data changes
My Plugin Management using Zustand Store
For my Figma plugin, I needed a powerful typography management system, TypographyStore
, to handle fonts, weights, styles, and their relationships. I also have ColorStore
for creating colors and their aliases, and MiscStore
for managing things like sizes, radius, and more. On top of that, I’m working on many other features.
Here’s snippets from my TypographyStore
how Zustand made all of this possible with minimal code:
// typographyStore.js
import { create } from 'zustand';
import { fontWeightTokens } from '../lib/typography';
import { loadFont } from './helpers';
import { ClientStorageManager } from './ClientStorageManager';
// Create the store
export const useTypographyStore = create((set, get) => ({
// State
typography: {
family: {
primary: "Inter",
},
weights: fontWeightTokens,
styles: {
"Heading/H1": {
fontSize: 48,
lineHeight: { value: 56, unit: "PIXELS" },
letterSpacing: -0.5,
weights: {
medium: "Medium",
}
},
// Other styles...
}
},
hasChanges: false,
isInitialized: false,
// Actions for font management
updatePrimaryFont: async (fontFamily) => {
try {
await loadFont(fontFamily);
set((state) => ({
typography: {
...state.typography,
family: {
...state.typography.family,
primary: fontFamily,
},
},
hasChanges: true,
}));
return true;
} catch (error) {
console.error('Failed to load primary font:', error);
return false;
}
},
// Style management
updateStyle: (styleName, style) => {
set(state => {
const updatedStyles = { ...state.typography.styles };
if (style) {
updatedStyles[styleName] = style;
} else {
delete updatedStyles[styleName];
}
return {
typography: {
...state.typography,
styles: updatedStyles
},
hasChanges: true
};
});
},
// Persistence layer
saveStyles: async () => {
const { typography } = get();
await ClientStorageManager.set('TYPOGRAPHY_STYLES', typography);
set({ hasChanges: false });
},
loadStyles: async () => {
const styles = await ClientStorageManager.get('TYPOGRAPHY_STYLES');
if (styles) {
set({ typography: styles, hasChanges: false });
}
},
// Initialize the store
initialize: () => set({ isInitialized: true }),
}));
Using Zustand here how it helps my Plugin
- Complex Nested State - Typography involves multiple levels of data (fonts, weights, styles). With Context API, I was constantly struggling with immutable updates to deeply nested objects. Zustand's set function makes these updates clean and predictable.
- Asynchronous Font Loading - Loading fonts is asynchronous, which was a pain to handle with reducers. Zustand lets me create async actions directly in the store without middleware or thunks.
- Persistence Between Sessions - Figma plugins need to save and load state. The get() method gives me access to the current state from any action, making it simple to implement storage operations.
- Plugin Communication - Figma plugins communicate between UI and the main thread. Having a centralized store makes it easier to sync state changes with the Figma document.
The beauty of Zustand is how cleanly it integrates with React components:
function FontSelector() {
// Only subscribes to the specific state needed
const primaryFont = useTypographyStore(state => state.typography.family.primary);
const updatePrimaryFont = useTypographyStore(state => state.updatePrimaryFont);
return (
<select
value={primaryFont}
onChange={(e) => updatePrimaryFont(e.target.value)}
>
{/* Font options */}
</select>
);
}
Specific Benefits I've Experienced
After refactoring my Figma plugin to use Zustand:
- Code Reduction - My state management code shrunk by ~60%
- Faster Development - Adding new features became much quicker
- Better Performance - Components only re-render when their specific data changes
- Easier Debugging - Having all state and actions in one place made tracking issues much simpler
Conclusion
I restarted the project from scratch with a blank project files, refactoring all the code and setting up a proper structure to keep it manageable. I think this is the biggest plugin I’ve worked on so far, with so many features to manage and different contexts to handle.
WIP mock of Plugin I'm working on
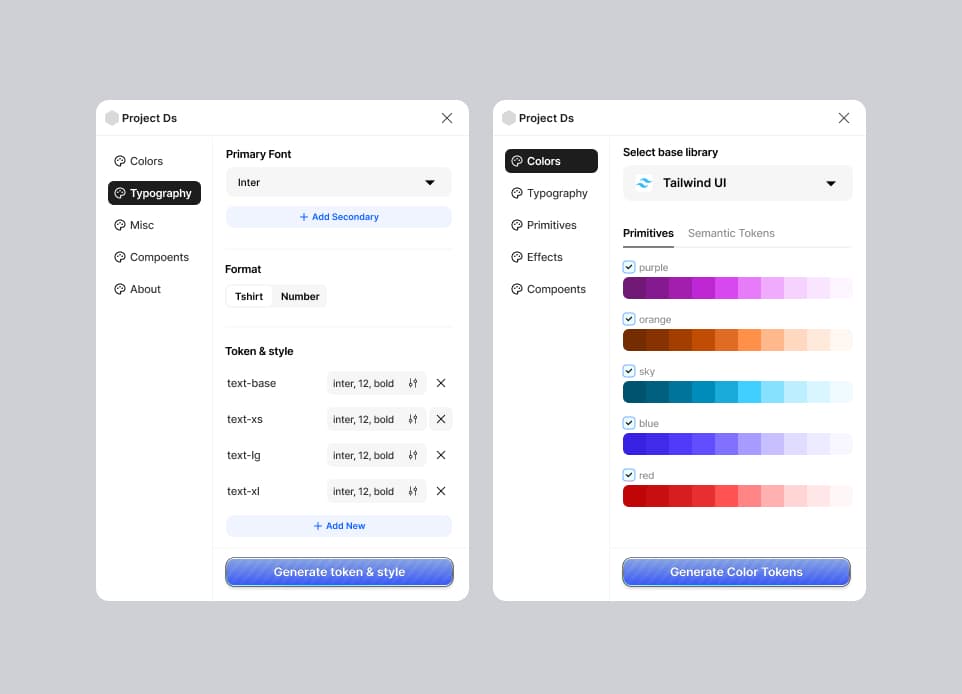
stay hungry, stay foolish
-Steve Jobs
©realvjy✦vijay verma